Adding autocomplete to address fields using the Google Maps JavaScript API can greatly improve the user experience on your website for users filling out forms. By suggesting valid addresses as users type, it can make it easier and faster to enter their address, reducing errors and frustration.
Using the Google Maps API also ensures that the addresses entered are accurate and properly formatted, helping to avoid common address-related errors. This can improve the accuracy and standardization of your data, making it easier to process and verify address information.
The end result should look like the address field in the form below. Type something to see how Google Maps autocompletes the address for you.
For this tutorial you will need:
- A Google Cloud account with billing details added.
- A form field where you want to autocomplete the address
- Access to add JavaScript to the page with the form.
Let’s get started!
Get the Google Places API Key
- If you have not yet enabled Google Maps JavaScript API, then log into your account for Google Cloud.
If you enabled it already, you can skip to step 5.
2) From the top-left corner, using the hamburger menu, navigate to APIs & Services.
3) Click on +Enable APIS AND SERVICES
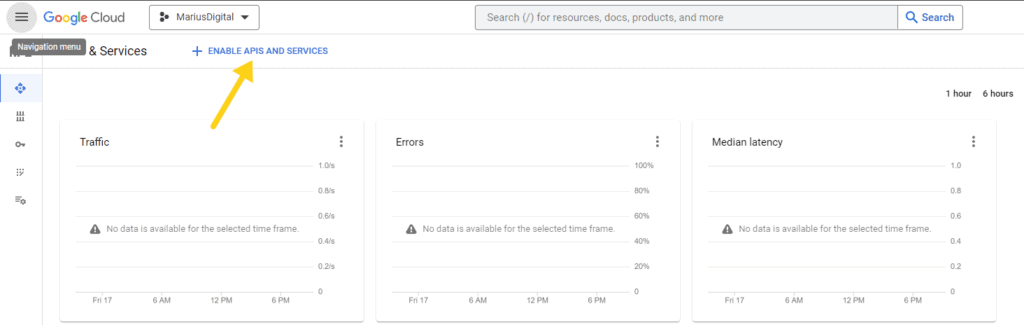
4) Select the Maps JavaScript API and Enable it.
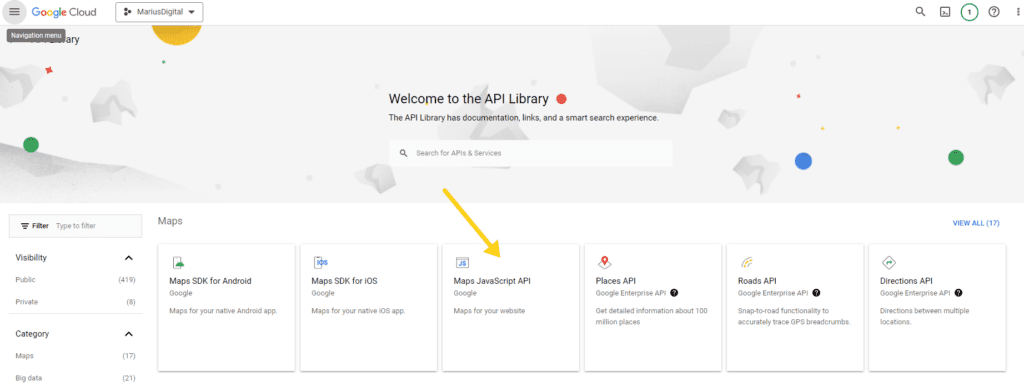
5) Get Your API Key.
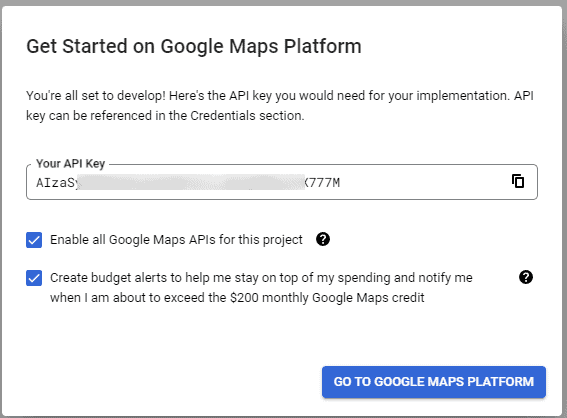
Load the Google Maps JavaScript API on your page
To load the Google Maps JavaScript API on the page(s), we need to add the following code snippet in the <head>
element of your page. Remember to replace YOUR_API_KEY
with the actual key you got at the previous step:
<script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&libraries=places&callback=initAutocomplete"
async defer></script>
Code language: HTML, XML (xml)
Alternatively, if the solution you are using to build the page does not allow editing the HTML to add the snippet above, but gives you access to insert custom JavaScript only (many page builders do this), then you can achieve the same result with the following JS code snippet:
var insertGoogleMapsApi = document.createElement("script");
insertGoogleMapsApi.type = "text/javascript";
insertGoogleMapsApi.defer = "true";
insertGoogleMapsApi.src = "https://maps.googleapis.com/maps/api/js?callback=initAutocomplete&&libraries=places&key=YOUR_API_KEY";
document.head.appendChild(insertGoogleMapsApi);
Code language: JavaScript (javascript)
Remeber to replace YOUR_API_KEY
with your actual API Key.
Do not use both snippets from above. Either one will work, based on what access you have on that page.
Add Google Places autocomplete to your form
Now, Google’s API is ready to autocomplete our form fields. We just have to specify which field.
Each field in a form should have a unique name attribute. Most form builders will allow to either edit it, or at least show it for each field. I built the demo form on this page using Fluent Forms and I’ve set this field’s name to form_address
.
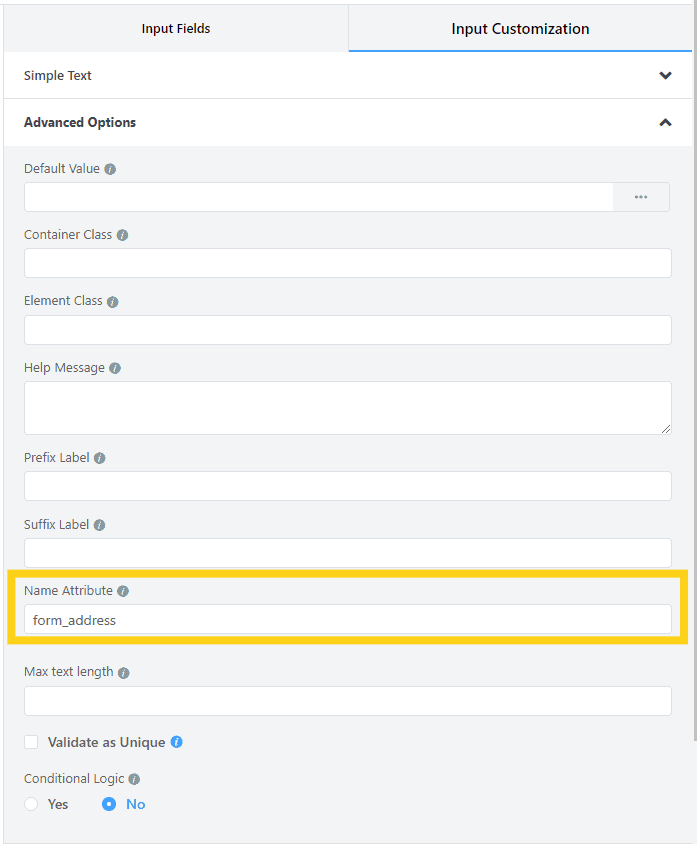
Once you know your field’s name attribute, you will need to add the following JavaScript code snippet to your page.
function initAutocomplete() {
// Create the autocomplete object
var input = document.querySelector('input[name="form_address"]');
var autocomplete = new google.maps.places.Autocomplete(input, {
types: ['geocode'],
componentRestrictions: { country: 'us' }
});
// Add listener to capture the selected place
autocomplete.addListener('place_changed', function() {
var place = autocomplete.getPlace();
// Optional: Do something with the selected place
console.log(place);
});
Code language: JavaScript (javascript)
If your form field has a different name, remember to replace form_address
` in the snippet above to your actual field’s name.
In addition, my example above restricts the autocomplete suggestions to the US only.
- If you want it to work globally, you can completely remove this line:
componentRestrictions: { country: 'us' }
- If you want the autocomplete to work for a specific country only, then you have to replace
us
with your desired country’s code
That’s it! You should now have a form field that autocompletes with suggestions from Google Places.
Tip if you have problems with the browser autofilling in a bad address on the form. Passwords won’t be autofilled.
jQuery(document).ready(function(){
setTimeout(function() {
jQuery(‘#input_1_30’).attr(‘autocomplete’,’new-password’);
}, 2000);
});